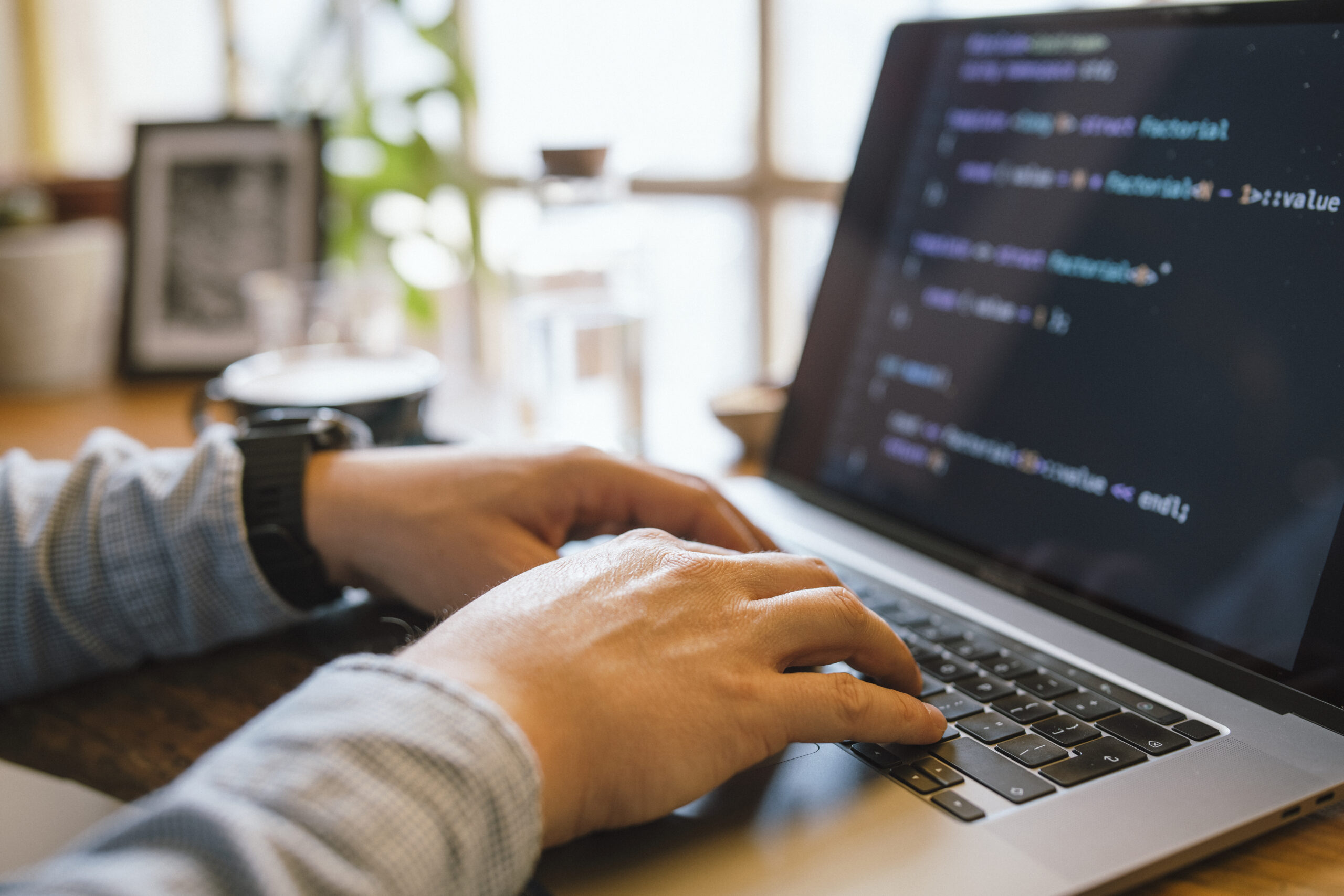
Debugging is Probably the most vital — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It's not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Assume methodically to unravel challenges competently. Whether you are a starter or even a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically boost your productiveness. Here's many approaches to help you developers level up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches developers can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Component of development, recognizing tips on how to communicate with it successfully in the course of execution is equally vital. Modern enhancement environments appear equipped with powerful debugging abilities — but numerous developers only scratch the area of what these instruments can do.
Choose, by way of example, an Integrated Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage via code line by line, and perhaps modify code about the fly. When utilized the right way, they Allow you to notice particularly how your code behaves in the course of execution, which happens to be invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-conclusion developers. They enable you to inspect the DOM, monitor network requests, perspective genuine-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can switch frustrating UI troubles into workable duties.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle over working procedures and memory administration. Learning these resources could possibly have a steeper Discovering curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with version Manage techniques like Git to understand code background, locate the precise minute bugs ended up released, and isolate problematic adjustments.
Eventually, mastering your instruments suggests likely further than default configurations and shortcuts — it’s about developing an intimate understanding of your growth setting making sure that when challenges crop up, you’re not shed at midnight. The better you realize your resources, the more time you can spend solving the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most critical — and often overlooked — steps in helpful debugging is reproducing the condition. In advance of jumping in to the code or generating guesses, developers need to produce a reliable setting or situation where the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, generally resulting in wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Ask concerns like: What steps resulted in the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it will become to isolate the exact disorders beneath which the bug occurs.
As you’ve collected more than enough details, try to recreate the challenge in your neighborhood ecosystem. This could signify inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem appears intermittently, take into account writing automated checks that replicate the edge circumstances or point out transitions concerned. These checks not only support expose the condition but in addition protect against regressions in the future.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on certain working systems, browsers, or below distinct configurations. Applying tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Using a reproducible situation, You may use your debugging applications more successfully, check prospective fixes securely, and talk additional Plainly with the staff or end users. It turns an abstract grievance into a concrete challenge — and that’s in which developers prosper.
Browse and Have an understanding of the Mistake Messages
Error messages are frequently the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really study to take care of mistake messages as direct communications in the system. They generally inform you just what happened, exactly where it happened, and from time to time even why it took place — if you know how to interpret them.
Get started by looking at the message carefully and in whole. Several builders, particularly when below time tension, glance at the first line and immediately start out producing assumptions. But further while in the error stack or logs may possibly lie the accurate root induce. Don’t just duplicate and paste error messages into search engines — read through and comprehend them initially.
Break the mistake down into parts. Can it be a syntax error, a runtime exception, or simply a logic error? Will it point to a certain file and line number? What module or operate induced it? These thoughts can guidebook your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging system.
Some glitches are imprecise or generic, and in Individuals conditions, it’s crucial to examine the context through which the mistake transpired. Test related log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These usually precede larger concerns and provide hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s happening under the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Popular logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through improvement, INFO for typical gatherings (like profitable commence-ups), WARN for potential difficulties that don’t split the appliance, ERROR for actual complications, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your method. Focus on critical activities, state improvements, enter/output values, and critical conclusion factors in your code.
Structure your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments where stepping by way of code isn’t possible.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging tactic, it is possible to lessen the time it will take to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and reliability within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently detect and repair bugs, builders will have to approach the process just like a detective fixing a thriller. This way of thinking allows stop working complex difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Look at the signs of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much relevant information as you can without having jumping to conclusions. Use logs, check instances, and user reports to piece together a transparent photo of what’s occurring.
Following, variety hypotheses. Check with on your own: What may very well be triggering this conduct? Have any adjustments lately been made towards the codebase? Has this challenge transpired in advance of beneath equivalent situations? The goal is to slender down options and discover prospective culprits.
Then, take a look at your theories systematically. Endeavor to recreate the challenge inside a managed natural environment. In case you suspect a specific functionality or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code questions and Permit the outcome lead you nearer to the truth.
Pay near interest to little aspects. Bugs typically hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or perhaps a race affliction. Be thorough and affected person, resisting the urge to patch The difficulty without having absolutely knowing it. Non permanent fixes could disguise the actual issue, just for it to resurface later.
And finally, continue to keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging process can preserve time for long term difficulties and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be more practical at uncovering hidden concerns in intricate units.
Create Exams
Producing checks is among the most effective approaches to increase your debugging expertise and Total enhancement performance. Tests not only aid catch bugs early but in addition function a security Internet that offers you self-confidence when producing alterations on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific piece of logic is Functioning as anticipated. Whenever a test fails, you immediately know where to glimpse, noticeably cutting down enough time put in debugging. Unit exams are Particularly helpful for catching regression bugs—issues that reappear just after Earlier currently being set.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These help be sure that a variety of elements of your software get the job done collectively smoothly. They’re specially beneficial for catching bugs that happen in elaborate programs with a number of components or products and services interacting. If anything breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically about your code. To test a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of being familiar with In a natural way leads to higher code composition and fewer bugs.
When debugging a difficulty, creating a failing exam that reproduces the bug may be a strong starting point. After the take a look at fails regularly, it is possible to focus on repairing the bug and enjoy your test go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—supporting you capture extra bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Option just after solution. But Probably the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new standpoint.
When you're too close to the code for too long, cognitive fatigue sets in. You might begin overlooking obvious errors or misreading code that you simply wrote just hours before. During this point out, your Mind results in being fewer economical at challenge-fixing. A short walk, a espresso crack, or maybe switching to a distinct activity for 10–quarter-hour can refresh your aim. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the background.
Breaks also enable avert burnout, Specifically throughout longer debugging periods. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, Gustavo Woltmann coding or possibly a misplaced variable that eluded you prior to.
In the event you’re trapped, an excellent general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, but it surely really brings about quicker and simpler debugging in the long run.
In a nutshell, having breaks just isn't an indication of weakness—it’s a wise technique. It offers your Mind space to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is a component of fixing it.
Master From Each and every Bug
Every bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you a thing important if you make the effort to replicate and review what went Incorrect.
Commence by asking by yourself some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit screening, code testimonials, or logging? The responses normally expose blind places as part of your workflow or knowledge and assist you Establish much better coding patterns going ahead.
Documenting bugs can be a fantastic routine. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see styles—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing Anything you've uncovered from a bug with your friends might be Specifically potent. Whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a much better Understanding culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, Every bug you deal with provides a fresh layer towards your skill established. So up coming time you squash a bug, have a moment to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging skills will take time, exercise, and patience — nevertheless the payoff is big. It will make you a more productive, self-assured, and able developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.